Added: 28 February, 2008
Group: PHP
Securing image uploads with PHP
Author: Alex
page: 1
Secure image uploads with PHP
Using PHP we can create special scripts that can check if user actually uploaded a picture or correct image size and dimensions.
When we are developing web pages that can let users to upload their pictures ti is advised to implement control of uploaded files how users won't use this form to upload something that is not a picture. This little security trick can save you a lot of time and bandwith.
First, lets create upload form:
<form action="action.php" method="post" enctype="multipart/form-data">
<input type="file" name="image">
<input type="submit" name="Submit" value="upload">
</form>
<input type="file" name="image">
<input type="submit" name="Submit" value="upload">
</form>
Notice that this for uses special HTML enctype attribute that determines how the form data is encoded. The value "multipart/form-data" should be used in combination with the INPUT element, type="file".
If you forget this part files won't be uploaded.
Now we should create action.php file that will process the image upload and security check.
Image should be defined with:
$_FILES["image"]["type"]
where image is the name of the form element which uploaded the image.
And new all we have to do is check if the uploaded file is image
if(($_FILES["image"]["type"]=="image/gif")OR($_FILES["image"]["type"]=="image/jpg")){
//if it is true upload file
}else{
//do not upload file contact administrator
}
//if it is true upload file
}else{
//do not upload file contact administrator
}
This little code checks if uploaded file is gif o jpg image and if it is true the file is uploaded. Otherwise warning message is displayed and file is not uploaded.
Of course this code can be modified to check for other image types and other warning messages.
Next step can be checking the size of uploaded image.
$_FILES["image"]["size"]
This code defines image and size element in the form. Checking the size of the uploaded file is managed with comparing to some size in bytes.
The checking code should look like this:
if($_FILES["image"]["size"]< 5000){
//if size is less than 5000 bytes upload
}else{
//do not upload file contact administrator
}
//if size is less than 5000 bytes upload
}else{
//do not upload file contact administrator
}
This code will upload the file if size is less that 5000 bytes and in other case upload will be canceled.
Upload of the file is managed with this php code:
$tmp_name = $_FILES["image"]["tmp_name"];
$location_to_server_location = "location/on/your/server";
move_uploaded_file($tmp_name, $location_to_server_location);
$location_to_server_location = "location/on/your/server";
move_uploaded_file($tmp_name, $location_to_server_location);
If you check these lines you might spot that it is advisable to use full pats in $location_to_server_location
Combining everything can be written in this way:
if((($_FILES["image"]["type"]=="image/gif")OR($_FILES["image"]["type"]=="image/jpg"))AND($_FILES["image"]["size"] < 5000)){
$tmp_name = $_FILES["image"]["tmp_name"];
$location_to_server_location = "location/on/your/server";
move_uploaded_file($tmp_name, $location_to_server_location);
}else{
echo "File is not image or it is larger than 5000 bytes ";
}
$tmp_name = $_FILES["image"]["tmp_name"];
$location_to_server_location = "location/on/your/server";
move_uploaded_file($tmp_name, $location_to_server_location);
}else{
echo "File is not image or it is larger than 5000 bytes ";
}
This small code will check if uploaded file is gif or jpeg image with size less than 5000 bytes.
This basic example explains most important elements of php code we use in uploading and checking the files in the form.
All of that can be altered and expanded to fit your special needs in image file uploads.
Enjoy in your play!
GO to: Page 1 : Secure image uploads with PHP
TechTut.com This tutorial is copyrighted. Partial duplication or full duplication is prohibited and illegal. Translation or usage of any kind without author�s permission is illegal.
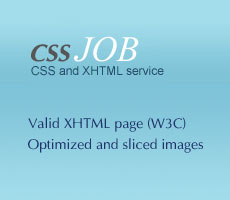

Use our RSS feed to get the latest published tutorials directly to your news reader.


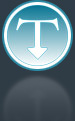
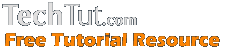